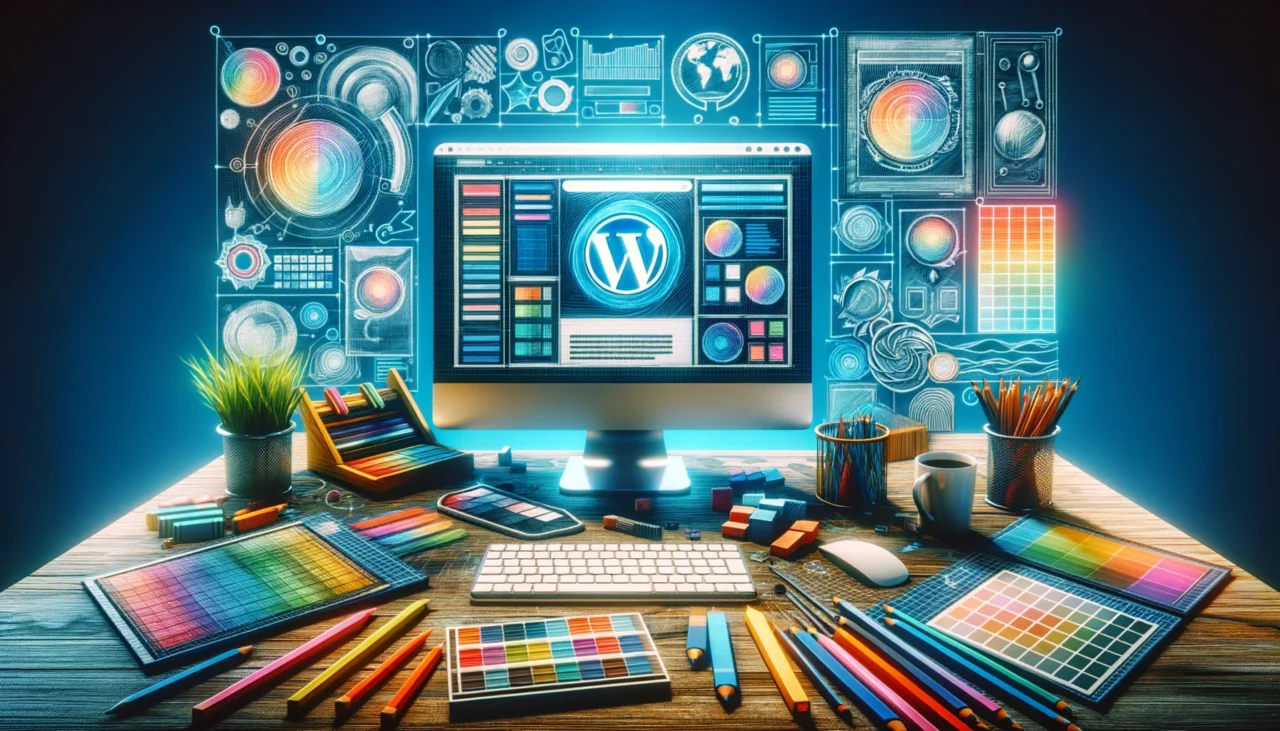
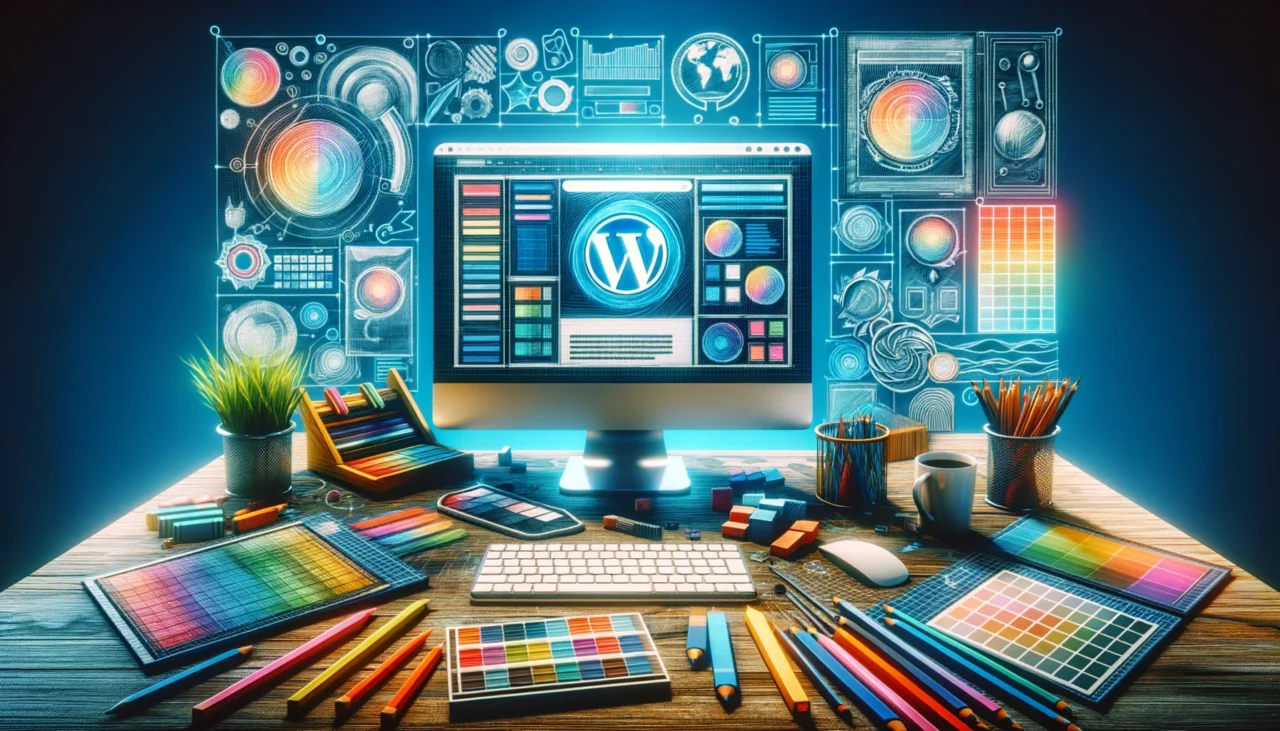
As a WordPress developer of 20 years, I’ve come to appreciate the power and flexibility that Custom post types and custom taxonomies bring to WordPress themes and plugins.
They are the secret weapons that transform a simple blog into a fully-fledged content management system, allowing for efficient management and content organisation. In this article, I will discuss custom post types and taxonomies, exploring their potential and how they can revolutionise your WordPress experience.
Understanding Custom Post Types
In the WordPress universe, everything is considered a ‘post’. Whether it’s a blog post, a page, or an attachment, they all fall under the umbrella of ‘post types’. By default, WordPress comes with several post types. However, to truly harness the power of WordPress, you can create your own Custom post types.
Custom post types are essentially content types that you define. They allow you to segregate different types of content, making it easier to manage and organise.
For instance, if you’re running a movie review site, you could create a Custom Post Type for ‘Movies’. This would allow you to separate your movie reviews from your regular blog posts, making your site more organised and easier to navigate.
Creating Custom Post Types
Creating a Custom Post Type in WordPress is relatively straightforward. You can do it manually by adding code to your theme’s functions.php file, or you can use a plugin like ‘Custom Post Type UI’. Here’s a simple example of how to create a Custom Post Type manually. This code creates a new Custom Post Type called ‘Movies’, which will now appear in your WordPress admin menu.
function create_posttype() {
register_post_type( 'movies', array(
'labels' => array(
'name' => __( 'Movies' ),
'singular_name' => __('Movie')
),
'public' => true,
'has_archive' => true,
'rewrite' => array(
'slug' => 'movies'
),
));
}
// Hooking up to theme setup
add_action('init', 'create_posttype');
Exploring Taxonomies
While Custom post types help you segregate your content, taxonomies help you classify it. In WordPress, a taxonomy is a way of grouping posts (or Custom post types) together. The default taxonomies in WordPress are ‘Categories’ and ‘Tags’, but you can create your own to further refine your content organization.
For instance, using our movie review site example, you could create a custom taxonomy for ‘Genre’. This would allow you to categorise your movie reviews by genre, making it easier for your visitors to find the type of movies they’re interested in.
Creating Custom Taxonomies
Creating a Custom Taxonomy is similar to creating a Custom Post Type. You can do it manually by adding code to your theme’s functions.php file, or you can use a plugin. Here’s an example of how to create a Custom Taxonomy manually. This code creates a new Custom Taxonomy called ‘Genre’, which you can now use to categorise your ‘Movies’ Custom Post Type.
function create_genre_taxonomy() {
$labels = array(
'name' => _x( 'Genres',
'taxonomy general name' ),
'singular_name' => _x( 'Genre',
'taxonomy singular name' ),
<span style="font-size: revert; background-color: initial; color: var(--ast-global-color-3);">);</span>
register_taxonomy( 'genre', array( 'movies' ), array(
'label' => __( 'Genre' ),
'hierarchical' => true,
'labels' => $labels,
));
}
add_action('init', 'create_genre_taxonomy',<span style="font-size: revert; background-color: initial; color: var(--ast-global-color-3);"> 0 );</span><p><span style="background-color: initial; color: var(--ast-global-color-3);"></span></p>
Enhancing WordPress Themes with Custom Post Types and Taxonomies
Custom post types and taxonomies can greatly enhance your WordPress themes by providing more control over your content. They allow you to create more complex content structures, making your site more dynamic and interactive.
For instance, you could create a ‘Portfolio’ Custom Post Type for a business theme, allowing businesses to showcase their work. Or you could create a ‘Products’ Custom Post Type for an e-commerce theme, allowing businesses to display their products.
Similarly, Custom taxonomies can enhance your themes by providing more specific categorisation options. For instance, for a news theme, you could create a ‘Location’ taxonomy, allowing news articles to be categorised by location.
Hire Me To Maintain & Improve Your Website
Take your website to the next level with affordable monthly maintenance plans to suit you.
Considerations When Using Post Types & Taxonomies
Optimising The Database
When it comes to database optimisation techniques, I try to focus on column indexing strategies to improve query performance. Creating indexes on frequently queried columns along with query caching and data restructuring have seen great results.
Regular database cleanup tasks and consideration of database sharding for scalability are also essential. Monitoring and profiling tools help in identifying and proactively addressing performance issues.
- Indexing strategies for faster queries
- Query caching and restructuring for improved performance
- Regular database cleanup tasks
- Consideration of database sharding for scalability
- Monitoring and profiling tools for proactive performance management
Optimising custom field data retrieval through meta query optimisation and selective indexing is crucial. Data normalisation and
Scaling for Growth
Horizontal scaling involves adding more servers or using load balancers to distribute traffic, while vertical scaling focuses on upgrading server resources. Caching mechanisms and queuing systems help in improving scalability and offloading server resources. Cloud-based auto-scaling solutions offer a flexible approach to handle traffic spikes.
- Horizontal and vertical scaling strategies
- Utilisation of caching mechanisms and queuing systems
- Adoption of cloud-based auto-scaling solutions
Implementing efficient caching strategies and queuing systems can enhance scalability. Utilising cloud-based auto-scaling solutions offers flexibility in resource allocation.
Enhancing Custom Field Efficiency
For custom field optimisation, I focus on meta query optimisation, selective indexing, and data normalisation to improve query performance. Lazy loading techniques and object caching help in reducing database load. It’s essential to consider efficient data retrieval and storage methods for custom fields.
- Meta query optimisation and selective indexing
- Database normalisation for well structured storage
- Lazy loading techniques to reduce unnecessary queries
- Object caching for improved performance
Optimising custom field retrieval and storage is crucial for improving query performance and reducing database load. Techniques like meta query optimisation and selective indexing enhanced performance.
Maximising Caching Mechanisms
Implementing object caching and page caching mechanisms help in reducing server load and improving response times. Cache invalidation strategies ensure that cached content remains up-to-date. Integrating a CDN further enhances caching performance.
- Object caching and page caching for reduced server load
- Cache invalidation strategies for up-to-date content
- Integration of CDN for enhanced caching performance
Object caching and page caching mechanisms improve server performance and response times. Utilising cache invalidation strategies and integrating CDNs further enhances caching efficiency.
Optimising Code Efficiency
Efficient query usage and minimising database calls are key to code optimisation. Utilising built-in WordPress functions and hooks and regular analysis of query performance help in identifying and addressing bottlenecks. Code reviews and collaboration with peers ensure adherence to best practices.
- Efficient query usage for improved performance
- Minimisation of database calls for reduced load
- Utilisation of built-in WordPress functions and hooks
- Regular analysis of query performance for optimisation
- Code reviews and collaboration for adherence to best practices
Efficient query usage and minimising database calls are crucial for code optimisation. Regular analysis of query performance and collaboration with peers help in identifying and addressing performance issues.
Enhancing User Experience
UI/UX considerations focus on designing user-friendly interfaces with accessibility in mind. Visual consistency and feedback mechanisms enhance user experience. Usability testing helps in gathering feedback and making iterative improvements.
- User-friendly interfaces with accessibility features
- Visual consistency for cohesive user experience
- Feedback mechanisms for enhanced interaction
- Usability testing for iterative improvements
Designing user-friendly interfaces with accessibility and usability in mind is crucial. Visual consistency and feedback mechanisms play a key role in enhancing user experience.
Ensuring System Security
Security measures include data validation, proper permissions and capabilities settings, and secure authentication mechanisms. Regular security audits and vulnerability assessments help in identifying and addressing potential risks. Security awareness and training promote a culture of security.
- Data validation and secure authentication for protection
- Regular security audits and vulnerability assessments
- Security awareness and training for proactive measures
Data validation, proper permissions settings, and secure authentication mechanisms are essential for maintaining security. Regular security audits and training promote awareness and proactive measures.
Effective Documentation & Maintenance
Comprehensive documentation and regular maintenance are essential for efficient management. Version control and regular audits help in tracking changes and identifying areas for improvement. Effective communication and collaboration ensure documentation accuracy and maintenance prioritisation.
- Comprehensive documentation for reference
- Regular maintenance for ongoing stability
- Version control and audits for tracking changes
- Effective communication and collaboration for accuracy
Comprehensive documentation and regular maintenance are crucial for efficient management. Version control and regular audits aid in tracking changes and identifying areas for improvement.
Are You Struggling To Maintain Your Website?
I offer affordable monthly maintenance plans that help maintain your website, add new features and provide support.
Did You Learn Something New?
Custom post types and custom taxonomies are powerful tools that can transform your WordPress experience. They provide a level of flexibility and control that is not possible with the default WordPress post types and taxonomies. By understanding and utilising these tools, you can create more organised, dynamic, and interactive websites.
What are your thoughts on Custom post types and taxonomies? Have you used them in your WordPress projects? Share your experiences and insights in the comments below.