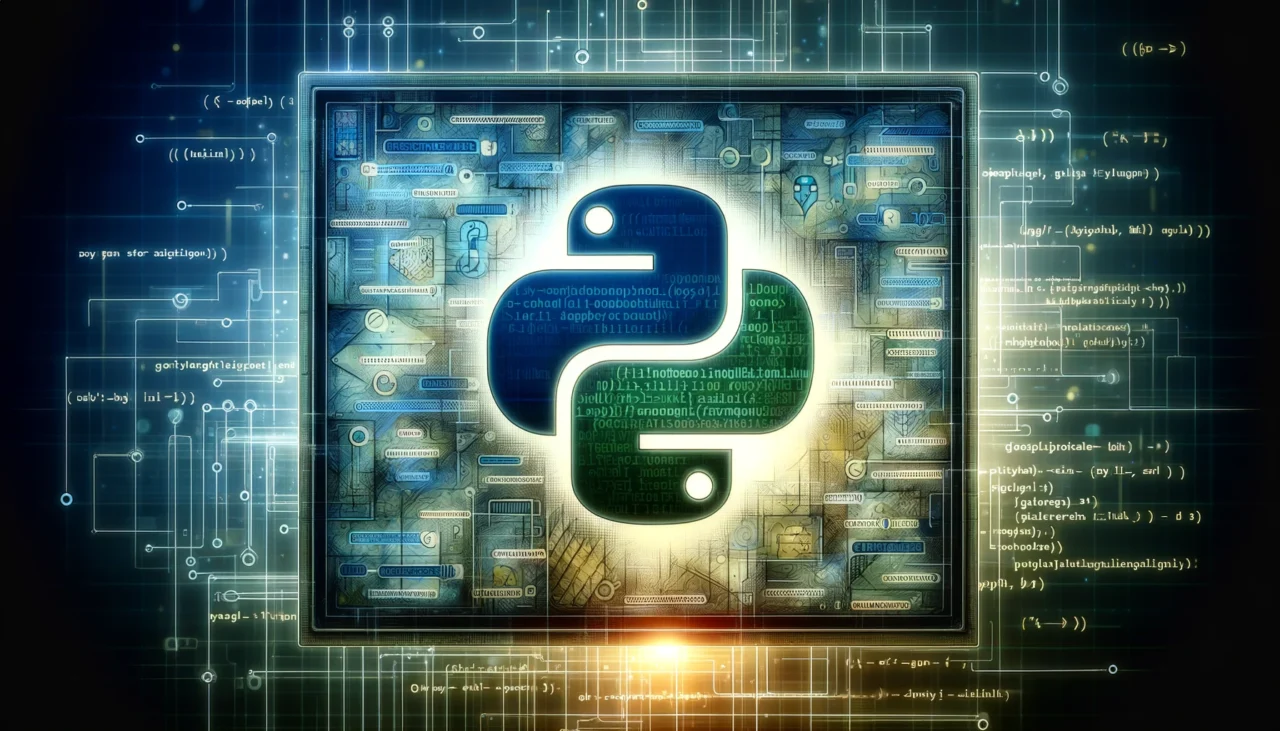
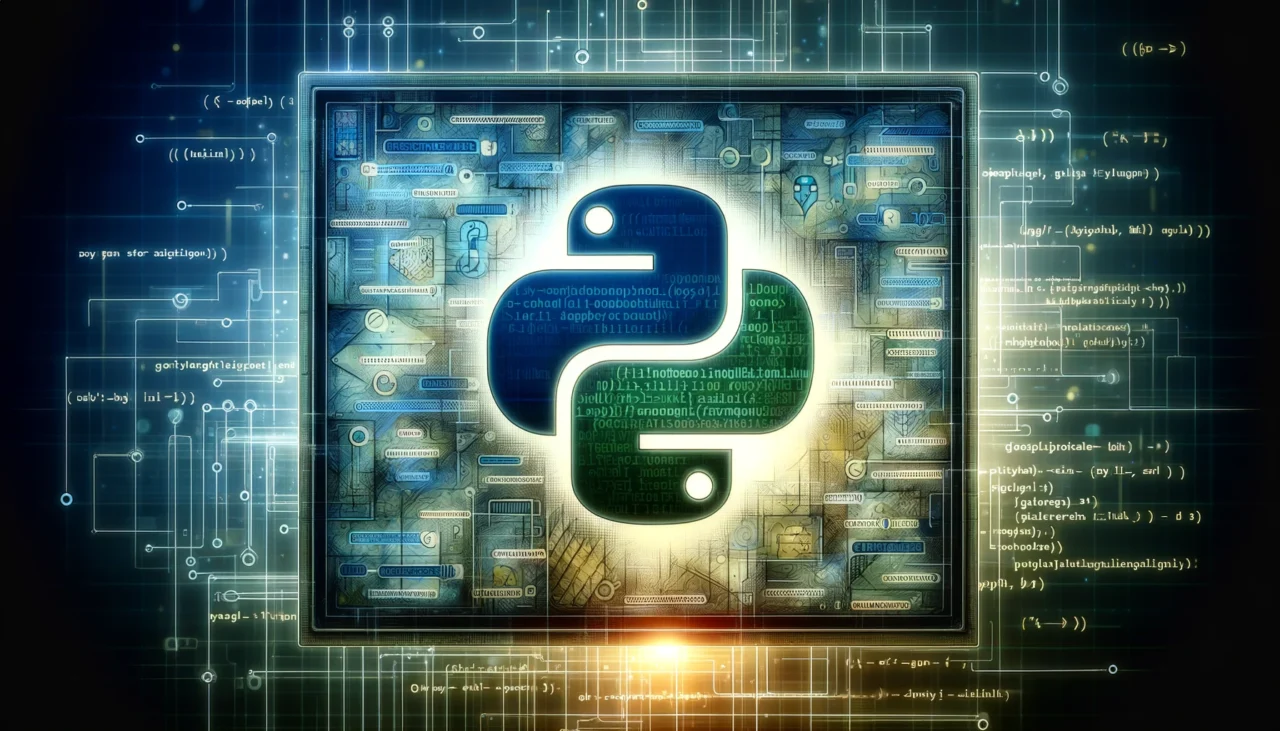
Automating tasks with Python transforms how we handle monotonous duties. With the right script, hours of work become minutes. Take web scraping as an example: it’s a clever way to gather data from websites.
You send requests and analyse HTML to pick out what you need, like prices or headlines. To begin automating such tasks in Python, make sure your toolkit’s complete – that means having Python installed alongside a good code editor like Visual Studio Code or PyCharm plus necessary libraries!
Before You Start
This guide is for those who know a bit about the Python Programming Language and be familiar with Python code syntax.
For clean code it is recommended to use Python classes and you should use the logging package for writing debug information to a log file. If (like me), you hate seeing logging statements across your code you can use a Python Decorator that acts as a wrapper around your function allowing you to perform tasks befor and after function execution.
It is always recommended to set up a virtual environment for your project to avoid library version conflicts. To do this please open a terminal window (command prompt on Windows) and run these commands:
python -m venv venv
source venv/bin/activate
BashWhen you want to use your script you must open your project directory in the terminal and run the source command above to load your project’s libraries and dependencies.
Automate Repetitive Tasks Using Python
You can use any Integrated Development Environment (IDE) that you choose, I am going to assume you are using VS Code (Free).
PyCharm is a dedicated Python code editor that provides a better experience over generic code editors, that you may want to consider using.
Python Web Scraping Code Example
In web scraping, I grab data from sites by asking for pages and looking at the HTML to get what’s needed. This info then goes into a database or file. Before running the script, ensure you have the necessary packages installed. You can install them using pip:
pip install requests beautifulsoup4
Pythonimport requests
from bs4 import BeautifulSoup
class Scraper:
def __init__(self, url):
self.url = url
self.soup = None
def fetch_content(self):
try:
response = requests.get(self.url)
response.raise_for_status() # Raises an HTTPError if the response status code is 4XX/5XX
self.soup = BeautifulSoup(response.content, 'html.parser')
except requests.exceptions.RequestException as e:
print(f"Request failed: {e}")
class BookScraper(Scraper):
def __init__(self, url):
super().__init__(url)
def parse_books(self):
if not self.soup: # Ensure the content has been fetched
print("Content not fetched. Please call fetch_content() first.")
return []
books = []
for book_div in self.soup.find_all("div", class_="book"): # Assuming each book is in a div with class 'book'
title = book_div.find("h3").text.strip() # Assuming the title is inside a <h3> tag
price = book_div.find("p", class_="price").text.strip() # Assuming the price is in a <p> tag with class 'price'
books.append({"title": title, "price": price})
return books
if __name__ == "__main__":
url = "https://example.com/books" # Replace with the actual URL
book_scraper = BookScraper(url)
book_scraper.fetch_content()
books = book_scraper.parse_books()
for book in books:
print(f"Title: {book['title']}, Price: {book['price']}")
PythonBest Practices Demonstrated:
Use of Classes: The script uses classes to encapsulate functionality, making it modular and reusable.
Exception Handling: Proper exception handling for HTTP requests to manage errors gracefully.
Separation of Concerns: Fetching content and parsing logic are separated into different methods for clarity and single responsibility.
Scalability: By using classes, it’s easy to extend this script. For example, you could add more methods to BookScraper for different parsing needs or create new scrapers by extending the Scraper class.
Python Email Automation & Notifications Code Example
In my years writing code, I’ve found that automating emails can be a big time-saver. Python’s smtplib module really shines here; it lets you send out many emails quickly. Imagine not having to manually type each individual email for your team or clients.
For emailing specifically, after setting up smtplib in your script, use an external file – say a CSV – where all recipient details are stored. Loop through this list while sending messages, personalising them if needed by plugging in names or other information from your data file.
# Filename: .env
# Email Credentis
EMAIL_HOST=smtp.example.com
EMAIL_PORT=587
EMAIL_ADDRESS=your_email@example.com
EMAIL_PASSWORD=your_password
Python# File Name: main.py
import csv
import smtplib
import os
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
from dotenv import load_dotenv
from time import sleep
# Load environment variables
load_dotenv()
class EmailSender:
def __init__(self):
self.host = os.getenv('EMAIL_HOST')
self.port = os.getenv('EMAIL_PORT')
self.email_address = os.getenv('EMAIL_ADDRESS')
self.password = os.getenv('EMAIL_PASSWORD')
self.server = None
def connect(self):
try:
self.server = smtplib.SMTP(self.host, self.port)
self.server.starttls()
self.server.login(self.email_address, self.password)
except smtplib.SMTPException as e:
print(f"Failed to connect or log in to the SMTP server: {e}")
raise
def send_email(self, recipient_email, recipient_name):
try:
msg = MIMEMultipart()
msg['From'] = self.email_address
msg['To'] = recipient_email
msg['Subject'] = 'Personalized Email Subject'
body = f"Hello {recipient_name},\n\nThis is a personalised message."
msg.attach(MIMEText(body, 'plain'))
# Pause for 5 minutes (as seconds) between sending each email
sleep(3600)
self.server.send_message(msg)
except smtplib.SMTPException as e:
print(f"Failed to send email to {recipient_email}: {e}")
finally:
del msg
def close_connection(self):
if self.server:
self.server.quit()
class CSVReader:
@staticmethod
def read_csv(file_path):
try:
with open(file_path, mode='r', newline='', encoding='utf-8') as file:
reader = csv.DictReader(file)
data = [row for row in reader]
return data
except FileNotFoundError:
print(f"The file {file_path} was not found.")
raise
except Exception as e:
print(f"An error occurred while reading {file_path}: {e}")
raise
def main():
try:
email_sender = EmailSender()
email_sender.connect()
recipients = CSVReader.read_csv('recipients.csv') # Assuming the CSV has columns 'Name' and 'Email'
for recipient in recipients:
email_sender.send_email(recipient['Email'], recipient['Name'])
except Exception as e:
print(f"An error occurred: {e}")
finally:
email_sender.close_connection()
if __name__ == '__main__':
main()
PythonBest Practices Demonstrated:
Exception Handling: The script includes try-except blocks to gracefully handle potential errors, ensuring the program doesn’t crash unexpectedly and provides useful error messages.
Environment Variables: Sensitive information like SMTP credentials is stored in environment variables, enhancing security by keeping them out of the source code.
Modular Design: The script is organised into classes and functions, promoting code reusability and maintainability by separating concerns (email sending and CSV reading).
Resource Management: The finally block ensures resources, such as the SMTP connection, are properly closed even if an error occurs, preventing resource leaks.
Scheduling Python Scripts
In this segment, we dive into the nuts and bolts of scheduling Python scripts. The tool for the job? Cron – it’s built right into Linux and macOS systems. With cron, I set up tasks that have to run on their own at set times or intervals – like every day or once a week.
So how does it work in practice? Imagine you want your script – let’s call it report_generator.py
to fire off a report every Monday morning without fail. You’d write a task to run in the crontab file for Linux and Mac users. You would use Scheduled Tasks within Windows systems, they all work in a similar way.
Mac and Linux Script Automation
To edit your crontab file on Linux and Mac type:
crontab -e
BashAdd a new line to the end of the file to schedule your script. For example, to run the script every day at 7 AM, you would add:
0 7 * * * /usr/bin/python3 /path/to/your/example_script.py 2>&1
BashThis is what tells cron exactly when to do its thing, if you need help writing Cron rules then the linked website can help generate the correct schedules. By using simple commands in the crontab, even complex jobs become automated, freeing you up from repetitive manual runs. It’s perfect for managing database cleanups or processing data overnight while most people sleep.
Windows Script Automation
Automatically Handling Files & Directories
In my two decades crafting words, I’ve never tired of showing people how to make their digital lives easier. Today, let’s talk about tidying up files with a bit of Python magic. Using the “os” library lets us sort out our messy folders in no time.
Here’s what happens: we use listdir()
to see what lurks inside our directory. You can think of it like peeking into a drawer full of odds and ends. Spot any ‘.pdf’ at the end? With one command we can move those to a folder or delete them without touching the Recycle Bin.
For those who love to use their desktop, the Pyautogui library is your friend here. It can type messages or move files around effortlessly! It is honestly amazing and automating these tasks feels like bringing a touch of wizardry to mundane life.
Database Management With Python
In my two decades of writing, I’ve seen Python revolutionise task automation. Its simplicity is unmatched; think plain English but with superpowers. For database management, this ease becomes invaluable.
Begin by installing Python on your system. Once that’s sorted, you just name your file main.py and open your code in your development editor. Now to run it: pop open Terminal on Mac or Command Prompt if you’re using Windows. Just type “python3”, add your script’s file name and hit enter.
Data Clean Up & Processing
With Python automation, scripts do magic. They sort through piles of data, they make sense out of chaos. I’ve seen firsthand how a few lines changed hours’ worth work into minutes’. Now take automated reporting – it’s less about grunt work more about insights now.
- Pandas: A powerful library for data manipulation and analysis, providing data structures like DataFrames and Series, with extensive functions to clean, filter, and transform datasets efficiently.
- NumPy: Essential for numerical computations, offering multi-dimensional arrays and matrices, along with a vast collection of mathematical functions to operate on these arrays, aiding in data cleaning by handling numerical data operations.
- Matplotlib: A plotting library that allows for the creation of static, interactive, and animated visualisations in Python, making it easier to spot outliers, trends, and patterns in data.
- Seaborn: Built on top of Matplotlib, Seaborn extends its functionalities, providing a high-level interface for drawing attractive and informative statistical graphics, which can be crucial for data cleaning by visualising the distribution of data and identifying anomalies.
- Scikit-learn: Although primarily known for machine learning, it includes various preprocessing methods like scaling, normalisation, and imputation, which are essential for cleaning data before analysis or model training.
- Beautiful Soup: Useful for web scraping tasks, it helps in parsing HTML and XML documents, making it easier to extract clean data from web pages.
- OpenPyXL: A library to read/write Excel 2010 xlsx/xlsm/xltx/xltm files, it’s useful for cleaning and manipulating datasets stored in Excel files directly from Python.
When people ask me if Python can automate some of their day-to-day tasks, my answer is typically a big yes! From crunching numbers to testing web apps seamlessly with Selenium. Python doesn’t just ease tasks, it redefines the possibilities on what you can create.
Learning Python isn’t tough either, there are tons of resources out there to learn. A course might be all you need to start creating powerful tools yourself! So really, when we think Python automation in 2024: it’s not just coding skill, it’s crafting smarter ways to get things done.
Understanding Python Automation Basics
“In my years of writing code, I’ve found Python’s simplicity ideal for automating tasks. Think about a script like an assistant who does jobs without you telling them each step”
Ben Lacey – Senior Python Developer
Say goodbye to manual email sends; Python can handle this easily using the smptlib library – no extra installs needed! Fill PDFs or Excel files in a snap too with pdfrw or pdfplumber. Even web stuff gets simpler: need data from a website? Just hook up your script with the Requests library, send out a GET or POST command and boom – job done while you sit back. This isn’t just tech talk; learning these tricks could save you hours every week on routine computer chores!
Error Handling in Automated Tasks
In my years of scripting with Python, handling errors stands out as vital. Say a bit of code breaks during an automated task; you don’t want the whole script to crash too! We use something called ‘try-except blocks’ to catch these errors.
When I write scripts, they check for specific issues that could happen and deal with them right there – no stopping us! This keeps our automation smooth, even if things go south. If a web request fails due to no network connection, we don’t halt everything.We try again or log it for later review, maintaining consistent data flow without manual intervention.
Exploring Python Automation Libraries
Digging into advanced automation libraries, I’ve found Python a gem for tasks that seem dull or too complex. Remember when we’d write heaps of code? Look at how one line of Python does the trick – crafting secure passwords in seconds flat with a simple command!
Picture needing lots of screenshots; rather than click and save each one, there’s Selenium Webdriver to automate it all. Python 3 is brilliant really: the language nails simplicity while giving us tools for some serious work under the hood.
Wrapping things up, automating tasks with Python can reduce errors, improve performance and simplify your life. The language makes complex processes simple, saving time and hassle. It’s like having a digital assistant at your fingertips.
From mundane data entry to sorting emails, it takes the load off my shoulders.For anyone looking to streamline their workflow or dive into coding, I wholeheartedly recommend giving Python a try – its versatility in automation will not disappoint you!
Further Reading Resources
https://www.browse.ai/b/web-scraping-with-python
Automate Everything With Python: A Comprehensive Guide to Python Automation
Python Job Scheduling with Cron
https://www.kdnuggets.com/2022/11/3-useful-python-automation-scripts.html
https://medium.com/tech-talk-with-chatgpt/easily-automate-your-daily-tasks-with-python-a-free-alternative-to-paid-tools-b021b0b51880
https://zapier.com/blog/python-automation/https://www.learnenough.com/blog/automating-with-python
https://www.turing.com/kb/automating-tasks-with-python-scripts
https://www.lighthouselabs.ca/en/blog/how-python-is-used-in-automation
Python in automation testing
https://blog.sentry.io/automate-your-boring-tasks-with-python/