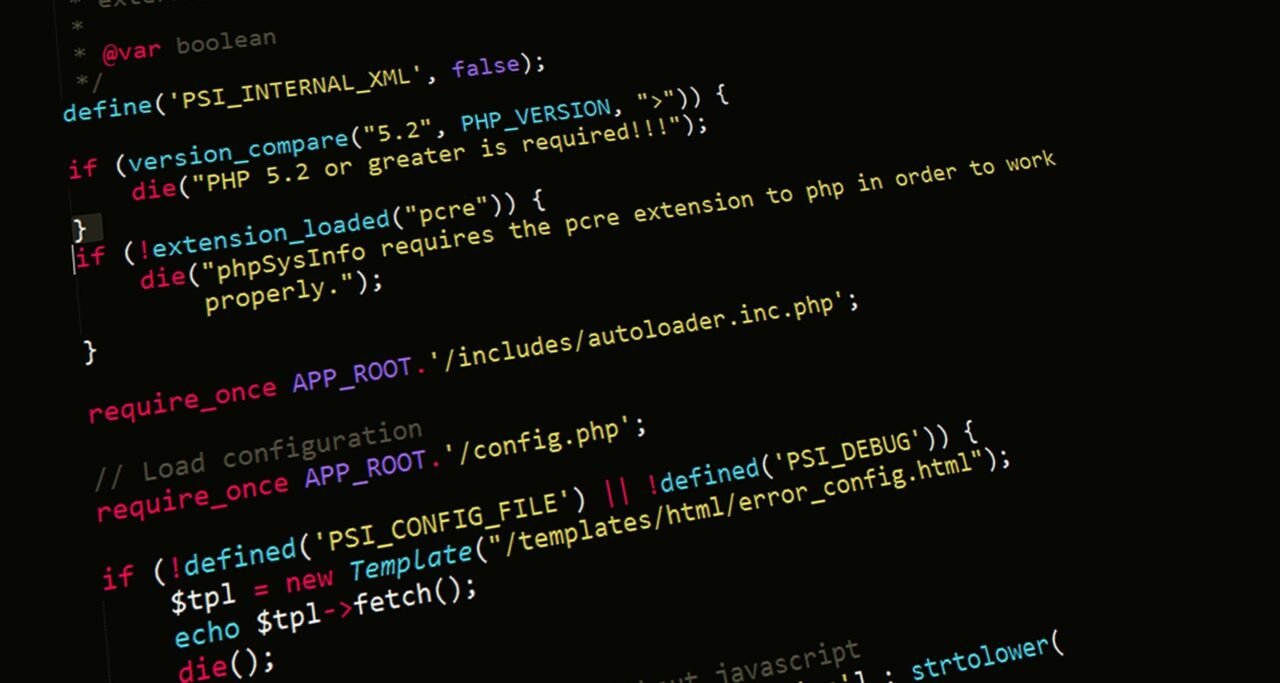
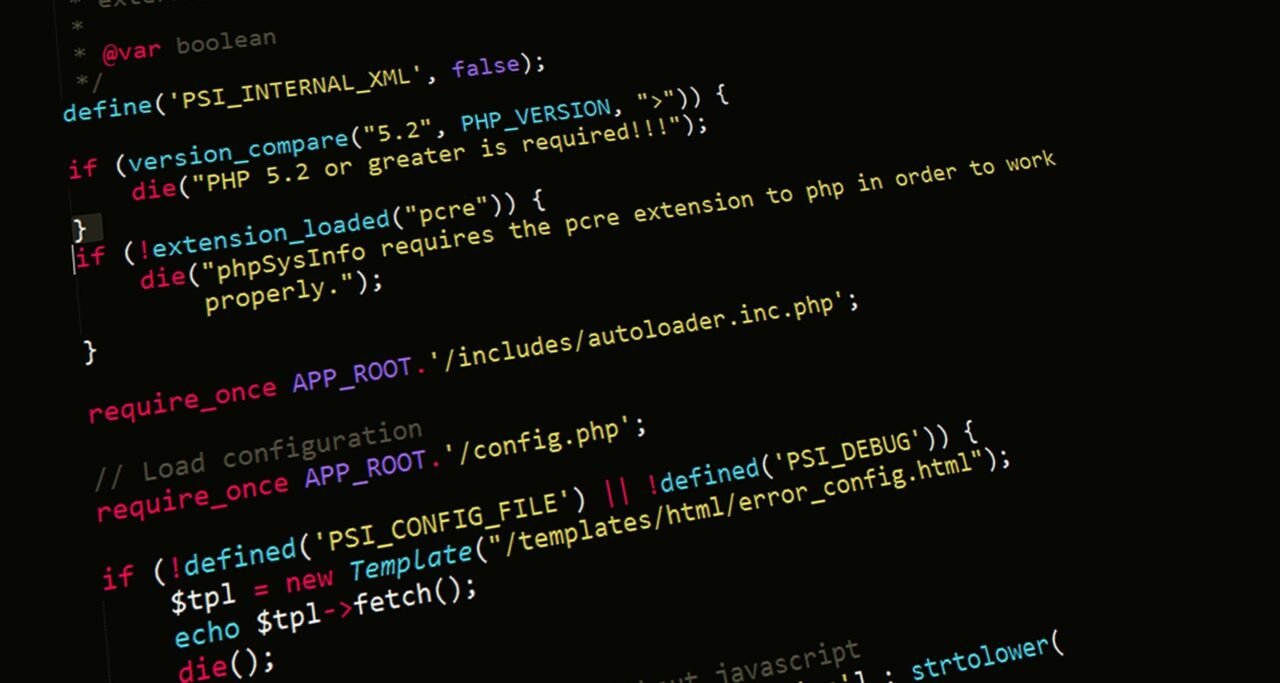
Laravel is an open-source PHP framework designed to make developing web applications easier and more efficient. It is based on the Model-View-Controller (MVC) architectural pattern and provides a robust set of tools and features to help developers create modern, feature-rich web applications.
Laravel is a great choice for web development because it offers a wide range of features and tools that make it easy to create powerful, dynamic web applications. It has a simple, expressive syntax that makes coding easier and faster. It also provides a number of helpful features such as routing, authentication, caching, and more. Additionally, Laravel is highly extensible, allowing developers to customise their applications to meet their specific needs.
Laravel is also highly secure, making it an ideal choice for developing applications that require a high level of security. It also has a large, active community of developers who are constantly working to improve the framework and provide support to users. Laravel is an excellent choice for web development. It is easy to use, highly extensible, and secure, making it an ideal choice for developing modern web applications.
Laravel Getting Started Guide
Laravel is an open-source PHP web framework that is designed to make developing web applications easier and more efficient. It is a powerful tool that can help you create amazing websites and applications quickly and easily.
In this guide, we will provide you with an introduction to Laravel and the basics of getting started. We will cover topics such as setting up your development environment, creating your first project, and understanding the Laravel architecture. By the end of this guide, you will have a basic understanding of how to use Laravel and be ready to start building your own applications.
First, let’s take a look at the development environment you will need to get started. You will need a web server, such as Apache or Nginx, and a database, such as MySQL or PostgreSQL. You will also need to install PHP and the Composer package manager. Once you have these components installed, you can begin setting up your Laravel project.
Next, you will need to create a new project. You can do this by running the “composer create-project” command in your terminal. This will create a new directory with the necessary files and folders for your project. You can then configure your project by editing the .env file. This file contains all of the settings for your project, such as the database connection and other environment variables.
Once your project is set up, you can begin exploring the Laravel architecture. Laravel is based on the Model-View-Controller (MVC) pattern, which is a popular way of organizing code. The Model is responsible for interacting with the database, the View is responsible for displaying the data, and the Controller is responsible for handling user requests.
Finally, you can start building your application. Laravel provides a number of helpful tools and features to make development easier. These include routing, authentication, and templating. You can also use the Artisan command-line interface to create and manage your project.
Benefits of Laravel Web Development
Laravel is an open-source PHP web framework that is designed to make web development easier and more efficient. It is one of the most popular frameworks for web development, and it is used by many developers around the world. Laravel offers a variety of benefits that make it an ideal choice for web development projects.
First, Laravel is highly secure. It has built-in security features that help protect websites from malicious attacks. It also has a robust authentication system that allows developers to easily create secure user accounts. Additionally, Laravel has a built-in system for handling authorisation and access control. This ensures that only authorised users can access certain parts of the website.
Second, Laravel is highly scalable. It is designed to be able to handle large amounts of traffic and data. This makes it ideal for websites that need to handle a lot of traffic or data. Additionally, Laravel is designed to be easily extensible. This means that developers can easily add new features and functionality to their websites without having to rewrite the entire codebase.
Third, Laravel is highly customizable. It has a wide range of features and tools that allow developers to customise their websites to meet their specific needs. This includes the ability to create custom themes, add plugins, and integrate third-party services. Laravel’s powerful blade templating system and the various supported front-end technologies makes it easy to create dynamic and feature rich web applications.
Laravel is designed to be fast and efficient, which makes it ideal for websites that need to load quickly. Additionally, Laravel is designed to be easy to use. This makes it ideal for developers who are new to web development or who don’t have a lot of experience.
Laravel is an excellent choice for web development projects. It is highly secure, scalable, customisable, and efficient. Additionally, it is easy to use and has a wide range of features and tools that make it ideal for developers of all skill levels. For these reasons, Laravel is an excellent choice for web development projects.
Creating Custom Authentication Using Laravel
Creating a custom authentication system with Laravel is a straightforward process that can be completed in a few simple steps. With the help of Laravel’s authentication scaffolding, you can quickly and easily create a secure authentication system for your application.
The first step is to install the Laravel authentication scaffolding. This can be done by running the following command in the terminal:
php artisan make:auth
This command will create the necessary views and controllers for authentication. It will also create a migration file for the users table, which will be used to store user information.
The next step is to create the user model. This can be done by running the following command in the terminal:
php artisan make:model User
This command will create a User model in the app directory. This model will be used to store user information.
The third step is to create the authentication routes. This can be done by adding the following code to the routes/web.php file:
Auth::routes();
This will create the necessary routes for authentication.
The fourth step is to create the authentication controllers. This can be done by running the following command in the terminal:
php artisan make:controller AuthController
This command will create the necessary controllers for authentication.
The fifth step is to create the authentication views. This can be done by running the following command in the terminal:
php artisan make:view auth
This command will create the necessary views for authentication.
The sixth step is to create the authentication middleware. This can be done by running the following command in the terminal:
php artisan make:middleware AuthMiddleware
This command will create the necessary middleware for authentication.
The seventh step is to create the authentication guards. This can be done by running the following command in the terminal:
php artisan make:guard AuthGuard
This command will create the necessary guards for authentication.
The eighth step is to create the authentication policies. This can be done by running the following command in the terminal:
php artisan make:policy AuthPolicy
This command will create the necessary policies for authentication.
The ninth step is to create the authentication providers. This can be done by running the following command in the terminal:
php artisan make:provider AuthProvider
This command will create the necessary providers for authentication.
The tenth step is to create the authentication notifications. This can be done by running the following command in the terminal:
php artisan make:notification AuthNotification
This command will create the necessary notifications for authentication.
Once all of these steps have been completed, you will have a fully functional custom authentication system with Laravel. With this system, you can easily and securely authenticate users and protect your application from unauthorized access.
Tips for Optimizing Performance in Laravel Applications
Use Caching: Caching is a great way to improve the performance of your Laravel application. By caching data, you can reduce the number of database queries and improve the response time of your application.
Use Efficient Database Queries: When writing database queries, make sure to use efficient query structures and avoid unnecessary joins. Also, use eager loading to reduce the number of queries needed to fetch data.
Use a CDN: Content Delivery Networks (CDNs) can help reduce the load on your server by serving static content from a distributed network of servers.
Optimise Your Assets: Make sure to minify and compress your CSS and JavaScript files to reduce the size of your assets and improve loading times.
Use a Task Queue: Task queues can help you offload long-running tasks from your web server and improve the performance of your application.
Use a Reverse Proxy: Reverse proxies can help reduce the load on your web server by caching static content and serving it directly to the user.
Use a Load Balancer: Load balancers can help distribute the load across multiple servers and improve the performance of your application.
Monitor Performance: Make sure to monitor the performance of your application and identify any bottlenecks that may be causing performance issues.
Overview of Laravel Features
Laravel is the latest version of the popular open-source PHP framework. It brings a number of new features and improvements that make it easier to develop applications with Laravel. Here is an overview of the new features in Laravel.
First, Laravel introduces a new model directory. This directory is used to store all of the models for your application. This makes it easier to organize and manage your models, as well as making it easier to find them when you need them.
Second, Laravel introduces a new router. This router is designed to make routing easier and more efficient. It also allows you to define routes in a more concise and readable way.
Third, Laravel introduces a new migration system. This system makes it easier to create and manage database migrations. It also allows you to rollback migrations if needed.
Fourth, Laravel introduces a new authentication system. This system makes it easier to authenticate users and manage their access to your application.
Finally, Laravel introduces a new job system. This system makes it easier to manage and schedule jobs in your application. It also allows you to queue jobs and run them in the background.
Overall, Laravel brings a number of new features and improvements that make it easier to develop applications with Laravel. These features make it easier to organise and manage your models, route requests, manage database migrations, authenticate users, and manage jobs.
How to Create a RESTful API with Laravel
Creating a RESTful API with Laravel is a straightforward process. Laravel is a powerful PHP framework that provides an easy way to create a RESTful API. It is designed to make developing, deploying, and maintaining applications much easier.
To get started, you will need to install the Laravel framework on your server. This can be done using the Composer package manager. Once installed, you will need to create a new project. This can be done using the Laravel command line interface (CLI).
Once the project is created, you will need to create the routes for your API. This is done in the routes/api.php file. Here, you will define the endpoints for your API. You can also define the HTTP methods that will be used for each endpoint.
Next, you will need to create the controllers for your API. This is done in the app/Http/Controllers directory. Here, you will define the logic for each endpoint. You can also define the response format for each endpoint.
Finally, you will need to create the models for your API. This is done in the app/Models directory. Here, you will define the database structure for your API. You can also define the relationships between the models.
Once all of the components of your API are in place, you can test it using a tool such as Postman. This will allow you to make sure that your API is working correctly.
Creating a RESTful API with Laravel is a straightforward process. With the right tools and knowledge, you can quickly create a powerful and secure API.
Exploring the Power of Laravel’s Eloquent ORM
Laravel’s Eloquent ORM is a powerful tool for developers, allowing them to quickly and easily interact with their database. It provides an expressive, fluent interface to working with the database, allowing developers to quickly and easily create, read, update, and delete records. Eloquent also provides powerful relationships, allowing developers to easily define relationships between their models.
Eloquent is an object-relational mapper (ORM), meaning it provides an abstraction layer between the database and the application. This abstraction layer allows developers to interact with the database using objects, rather than writing raw SQL queries.
This makes it easier to work with the database, as developers can use the same object-oriented programming techniques they are used to.
Eloquent also provides powerful relationships, allowing developers to easily define relationships between their models. This makes it easier to work with related data, as Eloquent will automatically handle the necessary SQL queries. Eloquent also provides powerful query builders, allowing developers to easily construct complex queries.
Eloquent has powerful caching capabilities, allowing developers to cache their queries and reduce the amount of time spent querying the database. This can significantly improve the performance of an application, as fewer queries need to be made.
Overall, Laravel’s Eloquent ORM is a powerful tool for developers, allowing them to quickly and easily interact with their database. It provides an expressive, fluent interface to working with the database, allowing developers to quickly and easily create, read, update, and delete records. Eloquent also provides powerful relationships, query builders, and caching capabilities, making it an invaluable tool for developers.
I hope this guide has given you a good introduction to Laravel and the basics of getting started. With the knowledge you have gained, you are now ready to start building your own applications. Good luck!