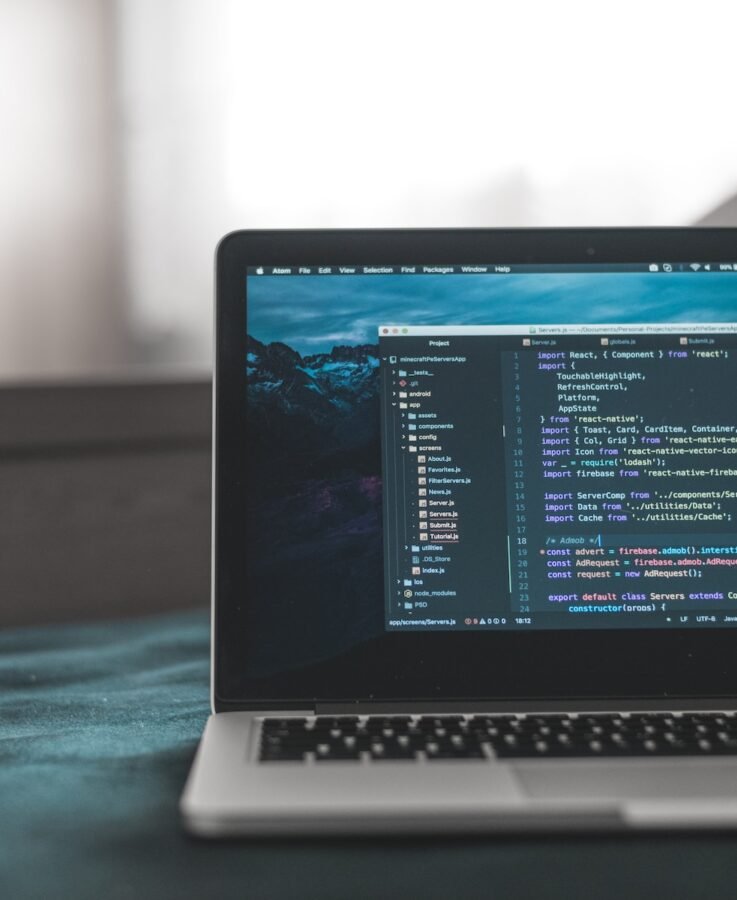
In the ever-evolving world of web development, PHP (Hypertext Preprocessor) has emerged as a powerful and widely-used scripting language. Its flexibility, ease of use, and extensive community support have made it a popular choice for building dynamic websites and web applications.
In this article, I will delve into the definition of PHP development, the importance of following best practices in PHP development and the current state of PHP development in 2023.
What Is PHP Development?
PHP development refers to the process of creating and maintaining web applications using the PHP programming language. Originally designed for server-side scripting, PHP has evolved into a full-fledged programming language capable of handling complex tasks. It allows developers to embed PHP code within HTML, making it seamless to generate dynamic content, interact with databases, and perform various server-side operations.
One of the key advantages of PHP is its cross-platform compatibility, enabling developers to deploy applications on different operating systems like Windows, Linux, and macOS. Moreover, PHP supports a wide range of databases, including MySQL, PostgreSQL, and Oracle, making it a versatile choice for database-driven applications.
Why Should You Follow PHP Development Best Practices?
Following best practices in PHP development is crucial for several reasons. Firstly, adhering to coding standards and conventions enhances the readability and maintainability of code.
Consistent indentation, meaningful variable names, and proper commenting not only make it easier for developers to understand the code but also facilitate collaboration among team members. PHP development best practices ensure the security of your code and web applications.
By implementing input validation, input sanitation and using MySQLi or PDO prepared statements when querying a database. PHP developers can protect against common vulnerabilities like SQL injection, Cross Site Request Forgery (csrf) and cross-site scripting (XSS) attacks from being successful.
Regularly updating your PHP version and libraries helps to fix security vulnerabilities and stay ahead of potential threats. Following best practices improves the performance of PHP applications.
Optimising code, minimising database queries, and utilising caching techniques (Redis, Memcache, File or localStorage) can significantly enhance the speed and efficiency of PHP web applications, resulting in a better user experience.
What Are PHP 8.2 Best Practices?
PSR Standards
Adhering to relevant PSR (PHP-FIG) standards for coding style, autoloading, and more. For example, PSR-12 specifies and enforces specific coding standards throughout your code base.
PHP-FIG (PHP Framework Interop Group) has established several standards, known as PSRs, to promote uniformity and best practices across PHP projects. PSRs cover various aspects of coding style, autoloading, logging, and more. Adhering to PSR standards enhances code consistency, collaboration, and maintainability within the PHP community.
PSR-1 and PSR-2 focus on basic coding style and recommendations. They outline conventions for file naming, class naming, method naming, indentation, and more. These standards ensure that code is readable and understandable by developers from different backgrounds.
Setup Local Docker Environments
Utilising Docker for creating consistent development and deployment environments that is lightweight and easily sharable. Docker is a platform that allows you to develop, deploy, and run applications using containers.
Docker containers are lightweight, isolated environments that package everything needed to run an application, including code, runtime, libraries, and dependencies. Docker simplifies the process of setting up consistent development, testing, and production environments, reducing “it works on my machine” scenarios.
Docker Compose is a tool that enables defining and running multi-container applications. You can use a docker-compose.yml file to specify the services, networks, and volumes required for your application’s components to interact seamlessly.
Unit, Feature and Integration Testing
Testing is a critical aspect of software development that ensures your code behaves as expected and reduces the likelihood of introducing bugs. There are several levels of testing you should consider.
Unit Testing: This involves testing individual units or components of your code in isolation. Unit tests ensure that each piece of code works as intended. Use PHPUnit or other testing frameworks to write and run unit tests.
Feature Testing: Feature tests verify that a specific feature of your application works correctly. These tests simulate user interactions and are often written using tools like Laravel’s PHPUnit testing suite.
Integration Testing: Integration tests validate the interactions between different parts of your application, ensuring they work well together. This type of testing can help identify issues that arise from the integration of various components.
Continuous Integration
Continuous Integration (CI) is a development practice where code changes are frequently integrated into a shared repository. With CI, developers merge their code into the main codebase multiple times a day.
Automated tests are run to quickly identify integration issues, bugs, or conflicts. CI ensures that the codebase remains healthy and stable, making it easier to catch and fix problems early.
Tools like Jenkins, Travis CI, and CircleCI automate the CI process by running tests whenever new code is pushed to the repository.
Continuous Deployment
Continuous Deployment is an extension of Continuous Integration. Once code passes the automated tests, it’s automatically deployed to a production environment. This practice speeds up the delivery process and reduces the time between writing code and seeing it live.
To implement Continuous Deployment, you need a reliable and automated deployment pipeline. Tools like Docker, Kubernetes, and cloud-based solutions (AWS, Google Cloud, etc.) help automate and manage the deployment process.
Object Orientated Programming
Object-Oriented Programming (OOP) is a programming paradigm that revolves around organizing code into reusable and modular objects. OOP promotes the use of classes, objects, inheritance, encapsulation, and polymorphism to create more maintainable and scalable code.
Classes and Objects: Classes define blueprints for objects, while objects are instances of those classes. Objects hold data (properties) and can perform actions (methods).
Inheritance: Inheritance allows you to create a new class based on an existing one. The new class inherits properties and methods from the parent class, promoting code reuse.
Encapsulation: Encapsulation restricts access to certain properties or methods, allowing you to control how data is manipulated and ensuring data integrity.
Polymorphism: Polymorphism enables you to create methods that can accept multiple types of objects, making your code more flexible and adaptable.
Code Decoupling
Code decoupling involves separating different components of your application so that they are independent and can function without tight dependencies. This promotes modularisation, making code more maintainable, testable, and reusable.
Dependency injection is a key technique in code decoupling. By injecting dependencies (such as objects, services, or configurations) into classes instead of creating them within the class, you reduce the coupling between classes. This allows for easier testing, swapping out components, and overall flexibility.
For instance, consider a class that needs a database connection. Instead of creating the database connection within the class, you inject it:
class UserRepository
{
private $dbConnection;
public function __construct(
DatabaseConnection $dbConnection) {
$this->dbConnection = $dbConnection;
}
// ...
}
Composer Dependency Management
Composer is a powerful tool for managing PHP dependencies. It simplifies the process of including external libraries and packages in your project. Instead of manually downloading and including libraries, you define your project’s dependencies in a composer.json file.
Composer retrieves the necessary packages from online repositories, ensuring consistent versions across development environments. This greatly reduces the headache of dealing with dependency conflicts and outdated libraries. To use Composer, create a composer.json file with your dependencies, then run composer install to use the package.
{
"require": {
"monolog/monolog": "^2.0"
}
}
Type Declarations
Type declarations in PHP 8 and later help catch type-related errors during development and provide better understanding of code interfaces. Type declarations are crucial for writing robust, self-documenting code. You can use type declarations for function parameters, return types, class properties, and more.
function calculateTotal(int $quantity, float $price): float {
return $quantity * $price;
}
Scalar type declarations (int, string, float, bool) ensure that function inputs and outputs have the expected types. Union types allow you to specify that a parameter can be of one of several types. Return type declarations help developers understand what to expect from a function’s return value. Additionally, using declare(strict_types=1) enforces strict type checking for each file, which reduces potential type-related errors.
Null Coalescing Assignment Operator
The null coalescing assignment operator (??=) is a feature introduced in PHP 7.4. It’s a concise way to assign a default value to a variable only if the variable is currently null. This operator simplifies the process of assigning default values, especially when dealing with optional variables or values retrieved from external sources.
$user = getUserFromDatabase();
$user ??= getDefaultUser();
In this example, if $user is null, it will be assigned the value returned by getDefaultUser(). Otherwise, the existing value of $user remains unchanged.
Match Expression
Use the match expression for clearer and more concise switch-like logic.
$color = getColorFromInput();
$message = match($color) {
'red' => 'Stop!',
'green' => 'Go!',
'yellow' => 'Slow down.',
default => 'Unknown signal.'
};
Attributes (Annotations)
Leverage attributes to add metadata to classes, methods, or properties. They provide a cleaner alternative to docblocks for certain annotations.
#[Route('/api/user')]
class UserController {
// ...
}
Constructor Property Promotion
Simplify class constructors by promoting properties directly in the constructor declaration.
class User
{
public function __construct(
private string $name,
private int $age) {
// ...
}
}
Named Arguments
Improve code readability by using named arguments when calling functions.
sendEmail(
to: '[email protected]',
subject: 'Hello',
message: 'Hi there!'
);
Union Types
Combine multiple types using union types to specify more flexible input/output expectations.
function processInput(int|string $input): void {
// ...
}
Immutable Data Structures
Use immutable data structures to prevent unwanted changes and bugs caused by mutability.
class Point
{
public function __construct(
public float $x,
public float $y
){
// ...
}
}
Error Handling using Throwable
Catch both exceptions and errors by catching the Throwable interface.
try {
// Code that might throw an exception or error
} catch (Throwable $e) {
// Handle the error or exception
}
Strict Comparison
Use strict comparison (=== and !==) to avoid type coercion and unexpected behavior.
if ($value === 5) {
// Do something
}
Hey Bob,
Thanks for leaving a comment and taking the time to read my article. It’s possible that you may have encountered challenges or frustrations with some of the tools and I know first hand that not every tool is useful. However, it’s worth considering the broader positive impact they have had on PHP development as a whole.
I understand that you have concerns about PHP-FIG/PSR and composer, but it’s important to acknowledge that these tools have played a significant role in advancing PHP development practices. PHP-FIG/PSR has helped establish a set of guidelines and standards that promote interoperability among PHP frameworks and libraries. This in my opinion has improved code reusability and collaboration within the PHP community.
While it’s true that many of the best practices discussed in the post are applicable to other languages and object-oriented paradigms, it’s important to emphasise that PHP has made significant strides in recent years to catch up with these standards.
I would love to have a further discussion with you to understand your perspective and maybe talk about solutions you have found that might be a better recommendation for this article to make it a better resource.
Keep on coding!
Ben
In my opinion, PHP-FIG/PSR and composer are probably the worst things to ever happen to PHP. Most of your points are pretty standard across most modern languages and Object Orientated paradigms.